How to Set Up a Basic Python Development Environment
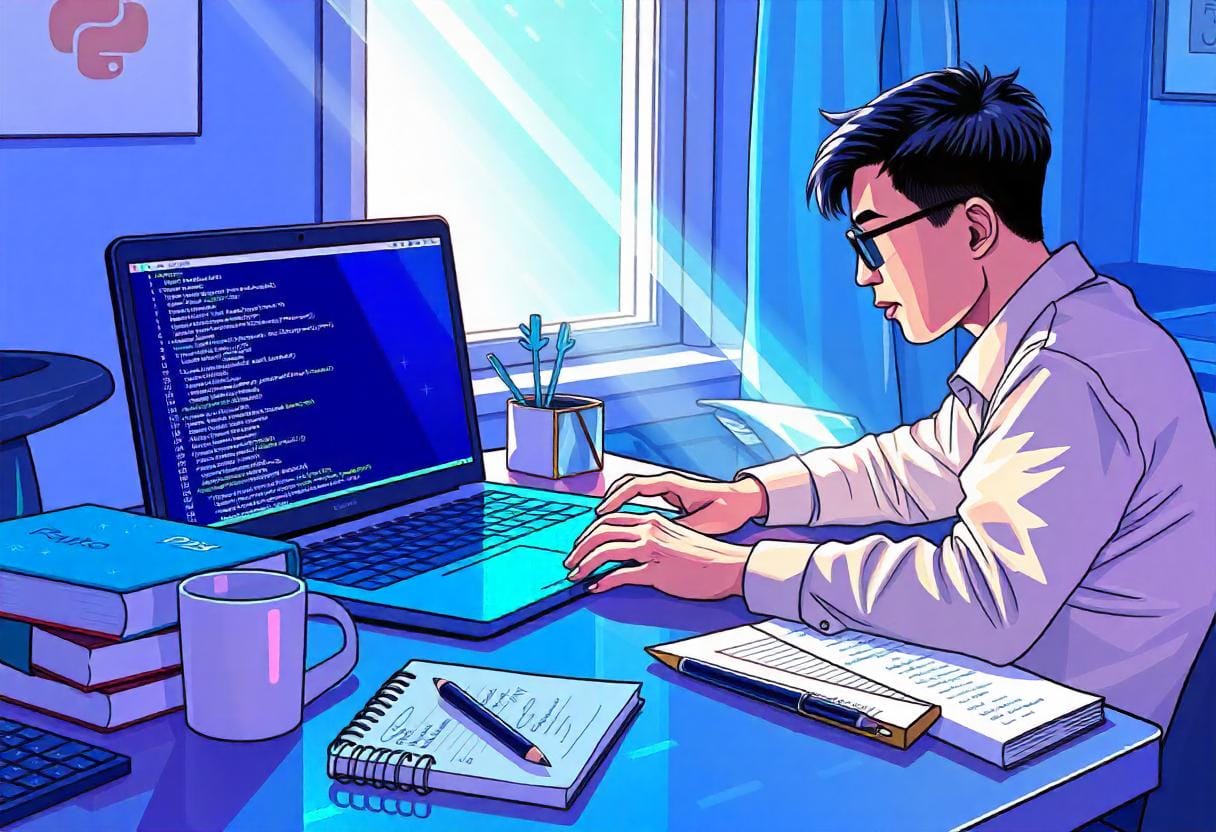
Python is one of the most popular programming languages, loved for its simplicity and versatility. If you're just starting out with Python, setting up the right environment can make your coding journey much smoother. Here's a simple guide to getting your Python development environment up and running.
Step 1: Install Python
First things first, you need to install Python on your machine.
- Go to the official Python website: https://www.python.org/
- Download the latest version: You’ll see a big "Download" button for your operating system (Windows, macOS, or Linux).
- Run the installer: Follow the prompts. Before clicking Install, be sure to check the box that says “Add Python to PATH.”
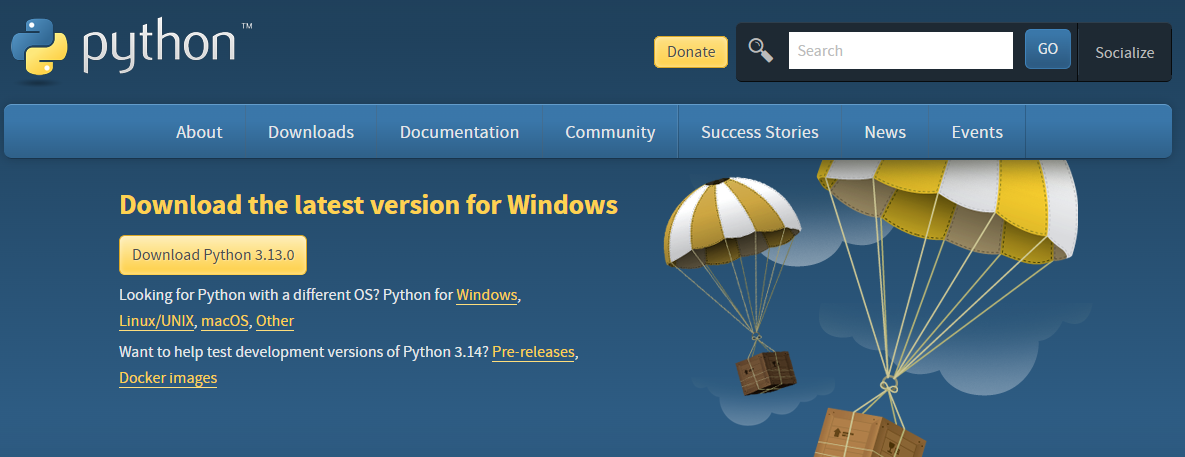
Step 2: Verify Python Installation
Once installed, you’ll want to check that everything went smoothly.
- Open your terminal (Command Prompt or PowerShell on Windows, Terminal on macOS or Linux).
- Type:
python --version
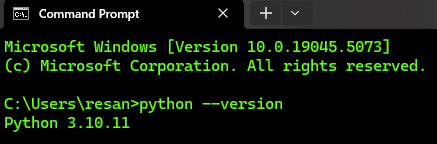
You should see the Python version you just installed.
Step 3: Install a Code Editor
While you can technically write Python in any text editor, a dedicated code editor will make your life easier. A great, free choice is Visual Studio Code (VSCode).
- Download VSCode: Visit https://code.visualstudio.com/.
- Install it and follow the setup instructions.
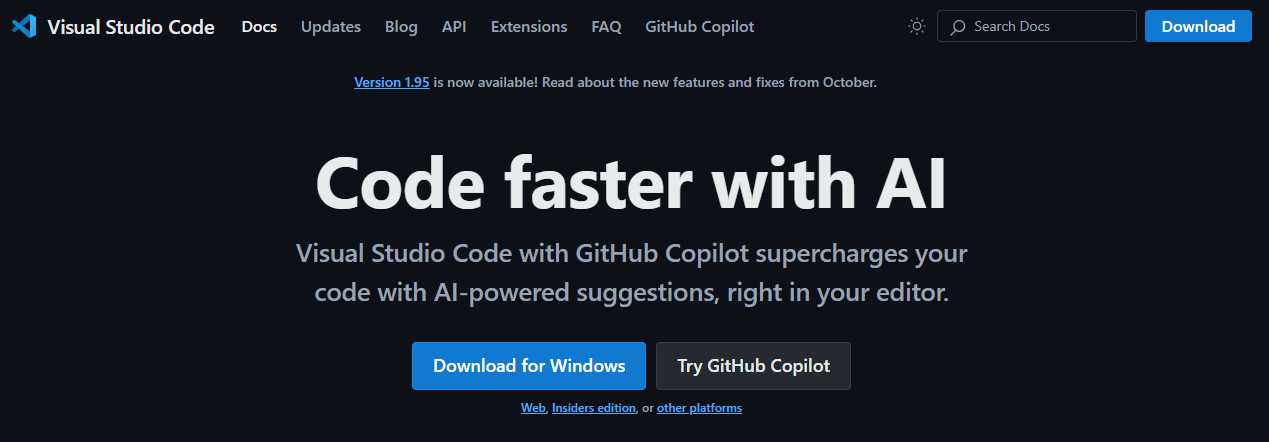
Once installed, open VSCode and install the Python extension to get syntax highlighting, IntelliSense and more.
Step 4: Set Up a Virtual Environment
A virtual environment helps organise your projects by allowing you to install dependencies (like libraries) for each project separately.
- Navigate to your project folder:
- Open your terminal and use
cd
to change to the folder where you want to store your Python project.
- Open your terminal and use
- Create a virtual environment:
python -m venv myenv
Replace "myenv" with your desired name for the environment.
- Activate the virtual environment:
- On Windows:
myenv\Scripts\activate
- On macOS/Linux:
source myenv/bin/activate
You should see (myenv)
in your terminal, which means the virtual environment is active.
Step 5: Install Libraries (Optional)
With your active virtual environment, you can install Python libraries to help with your projects. For example, if you’re working with web development, you might want to install Flask or Django.
To install a package, use the pip
command:
pip install flask
You can install multiple libraries at once by creating a requirements.txt
file, which lists all your dependencies.
Step 6: Run Your First Python Script
Now that your environment is ready, it’s time to write your first Python script!
- Open your code editor (VSCode).
- Create a new file with the extension
.py
(e.g.,hello_world.py
). - Type the following code:
print("Hello, World!")
- Save the file and run it in the terminal by typing:
python hello_world.py
You should see the output, Hello, World!
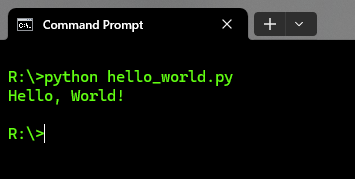
Conclusion
Congratulations! You've successfully set up a Python development environment. Now, you're ready to start writing more complex code and experimenting with Python's rich ecosystem. If you want to dive deeper, there are tons of resources available to help you expand your skills—keep coding!